JZZ.input.Knobs
Additional controls for your virtual piano. Support mouse and touch. Custom styles. Ready for responsive design.
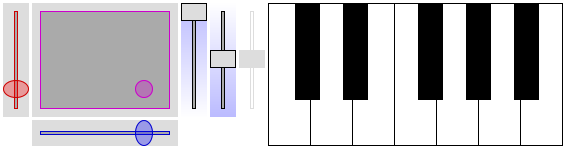
See the demo...
JZZ.input.Slider
JZZ.input.Slider(params);
// or
JZZ.input.Slider(name, params);
// or
JZZ.input.Slider.register(name, params);
JZZ().openMidiIn(name);
where params - is the object with the following possible keys:
-
at: the DOM element or the ID of the DOM element to host the keyboard;
if omitted, a new <DIV> element will be created in the end of the document.
-
pos: position; 'N' (default) - normal view; 'E' / 'S' / 'W'
- rotated by 90° / 180° / 270° clockwise.
-
kh and kw: knob height and width in pixels; default: 16 and 24.
-
rh and rw: range (the central area where the knob moves) height and width in pixels; default: 128 and 2.
-
bh and bw: containing box height and width in pixels; default: calculated from the knob and range dimensions.
-
chan: MIDI channel; default: 0.
-
data: MIDI data; see the details below.
-
base: base value; default: depends on the data type,
can be 0. as for modulation, or .5 as for balance.
-
val: initial value from 0. to 1.; default: equals to base.
-
active: if false, the control will not respond to mouse/touch, but it will still accept incoming MIDI messages.
-
onCreate: the function to run after the keyboard is created; it can be used for additional styling.
-
numeric values: responsive subparams; same as for JZZ.input.Kbd.
JZZ.input.Pad
A 2-dimentional cousin of JZZ.input.Slider
JZZ.input.Pad(params);
// or
JZZ.input.Pad(name, params);
// or
JZZ.input.Pad.register(name, params);
JZZ().openMidiIn(name);
where params - is the object with the following possible keys:
-
at: the DOM element or the ID of the DOM element to host the keyboard;
if omitted, a new <DIV> element will be created in the end of the document.
-
pos: position; 'N' (default) - normal view; 'E' / 'S' / 'W'
- rotated by 90° / 180° / 270° clockwise.
-
kh and kw: knob height and width in pixels; default: 16 and 24.
-
rh and rw: range (the central area where the knob moves) height and width in pixels; default: 128 for both.
-
bh and bw: containing box height and width in pixels; default: calculated from the knob and range dimensions.
-
chan: MIDI channel; default: 0.
-
dataX and dataY: MIDI data for horizontal and vertical movements; see the details below.
-
baseX and baseY: base values; same as above.
-
valX and valY: initial values; same as above.
-
active: if false, the control will not respond to mouse/touch, but it will still accept incoming MIDI messages.
-
onCreate: the function to run after the keyboard is created; it can be used for additional styling.
-
numeric values: responsive subparams; same as for JZZ.input.Kbd.
Data
Control can encode its value as a Pitch Bend message (Ex lsb msb),
or as one or two Control Change messages (Bx MM msb, Bx LL lsb),
where lsb/msb stand for the least/most significant byte,
and LL/MM are the corresponding control codes.
The data parameter can be:
-
a number: 0 for Pitch Bend, or MM for one-byte Control Change messages (Bx MM msb).
-
an array of two numbers: [MM, LL] for two-byte Control Change messages (Bx MM msb, Bx LL lsb).
-
a string shortcut for the most common MIDI controls:
-
'mod': Modulation Wheel; same as [0x01, 0x21].
-
'breath': Breath Controller; same as [0x02, 0x22].
-
'foot': Foot Controller; same as [0x04, 0x24].
-
'portamento': Portamento Time; same as [0x05, 0x25].
-
'volume': Channel Volume; same as [0x07, 0x27].
-
'balance': Balance; same as [0x08, 0x28].
-
'pan': Pan; same as [0x0a, 0x2a].
-
'expression': Expression Controller; same as [0x0b, 0x2b].
-
'effect1': Effect Control 1; same as [0x0c, 0x2c].
-
'effect2': Effect Control 2; same as [0x0d, 0x2d].
If the data parameter is missing or not recognized, defaults to Pitch Bend.
If both dataX and dataY parametes are skipped for the JZZ.input.Pad,
they default to Pitch Bend and Modulation Wheel.
Styling
Sliders and Pads can be customized by assigning styles and inner HTML for their
knob, range, or box DOM elements.
This can be done from the and(...) or onCreate(...) functions, or from any other part of your application at any time.
Functions that access the DOM elements are:
-
getKnob() - get the knob.
-
getRange() - get the range.
-
getBox() - get the box.
These elements can be modofied by the following functions:
-
setInnerHTML(string) - set the element's inner HTML.
-
setStyle(style1, style2) - apply style1 in the normal state, and style2 the knob is dragged by user.
-
setStyle(style) - apply style for both states.
Example
slider.getKnob().setStyle({borderRadius:'20%'})
.setStyle({backgroundColor:'rgba(0,0,255,0.5)'}, {backgroundColor:'rgba(255,0,0,0.5)'});
See also